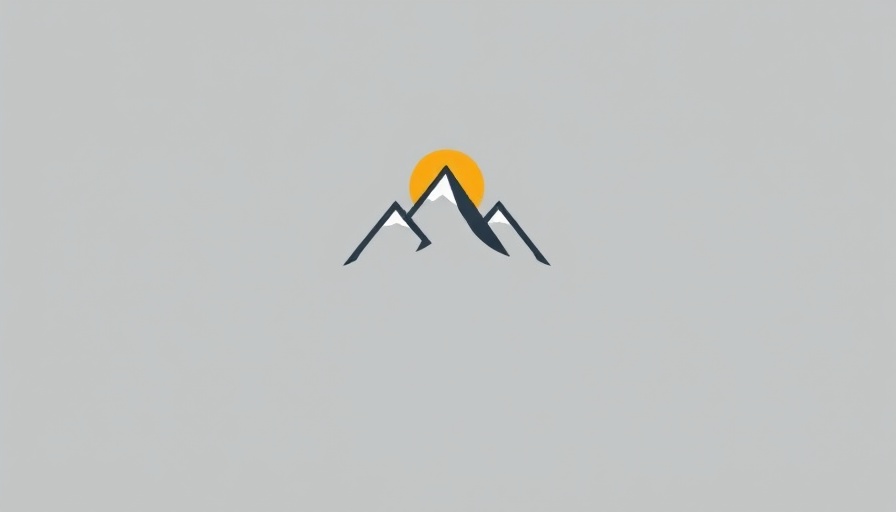
Unlocking the Power of Refs in React
React has revolutionized front-end web development with its efficient component-based architecture, enabling developers to create scalable and maintainable applications. One of the key features of React is the ability to manipulate and interact with DOM elements through refs. Understanding the nuances of refs not only helps in optimizing application performance but also elevates the user experience. In this article, we will explore advanced techniques to master refs, particularly focusing on the useCombinedRef
hook.
What Are Refs and Why Are They Important?
In React, refs are a way to directly interact with DOM elements. Typically used in forms and media playback, refs allow developers to bypass the usual data flow of props and state. This is especially useful for handling focus, text selection, or triggering imperative animations. However, misuse of refs can lead to issues with component reusability or state management. Hence, understanding advanced ref management techniques is crucial for React developers.
Introducing the useCombinedRef Hook
The useCombinedRef
hook stands out because it allows multiple refs to be combined into a single ref. This is particularly beneficial when you want to pass refs from different sources but still maintain a clear reference to your DOM element. Let's break down how this hook works with a simple code example.
import React, { useRef, forwardRef } from 'react'; function useCombinedRef(...refs) { return node => { refs.forEach(ref => { if (typeof ref === 'function') { ref(node); } else if (ref) { ref.current = node; } }); };
}
Implementing useCombinedRef: A Step-by-Step Guide
Now that we have an understanding of the useCombinedRef
hook, let's implement it within a functional component. This demonstration will highlight the simplicity and power of combining refs:
const MyInput = forwardRef((props, ref) => { const localRef = useRef(); const combinedRef = useCombinedRef(localRef, ref); return ;
});
This approach ensures that both the local ref and the forwarded ref are pointing to the same input DOM element, making it easier to manage focus or value changes across components.
Practical Applications of useCombinedRef
The benefits of using useCombinedRef
extend beyond simple DOM manipulation. Here are some practical scenarios where this hook can enhance your React applications:
- Complex Form Handling: When you have nested forms or multiple inputs that need validation, combining refs can streamline error handling.
- UI Component Libraries: If you're building a UI library, use refs to allow users to customize components while maintaining control over the underlying DOM.
- Animation Libraries: Combining refs can help in synchronizing animations across several components efficiently.
Common Misconceptions About Refs
Many developers believe that refs should be used frequently and replaced with state management. This ideology, however, can lead to problematic performance issues. Instead, refs should be leveraged where necessary, especially when there's a need for direct DOM manipulation which cannot be achieved through state.
Future Trends in React Development
The React ecosystem is continuously evolving, with hooks representing a significant shift in how developers approach component design. As libraries and frameworks around React mature, expect to see more sophisticated techniques for handling refs, including built-in hooks which encapsulate patterns like useCombinedRef
more naturally.
Conclusion
Mastering refs and advanced hooks like useCombinedRef
drives better React application performance, mitigates common pitfalls, and enhances the user experience. Embrace these techniques, and you'll find that your React applications become more powerful, scalable, and reusable than ever before.
Write A Comment