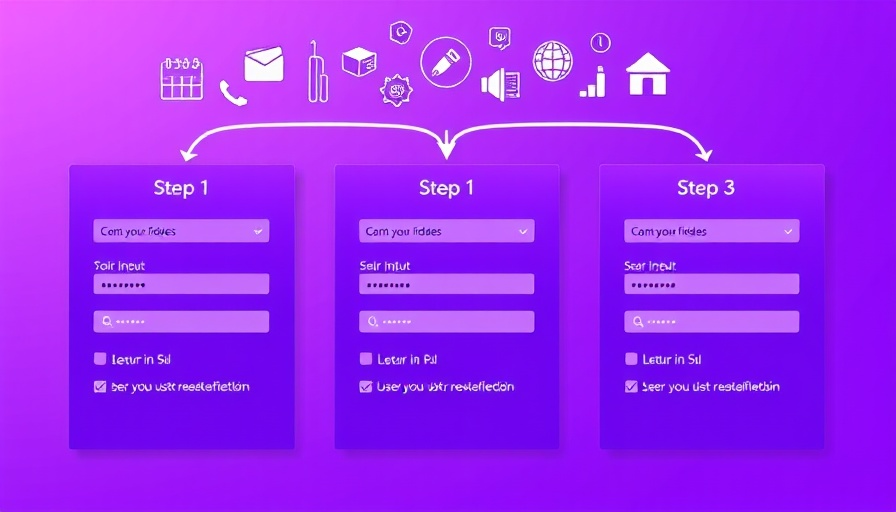
Why Building Multi-Step Forms Matters
In today's fast-paced digital landscape, forms remain a critical component of user interactions, whether for onboarding, checkout, or user feedback. Multi-step forms present an effective solution to managing extensive data entry processes. They streamline user interaction by breaking down complex forms into digestible segments, leading to increased completion rates and a more satisfying user experience. Instead of overwhelming users with long single-stage forms, breaking the information collection into manageable steps allows users to focus on one task at a time.
A Step-by-Step Guide to Creating Multi-Step Forms
This article will delve into the practicalities of crafting a reusable multi-step form using React Hook Form and Zod for validation. Designed with reusability in mind, this component not only enhances user experience but also simplifies development processes.
Key Prerequisites for Developers
Before embarking on this journey, ensure that you have a clear understanding of React's state management, its Context API, and some experience with React Hook Form. Familiarity with TypeScript will also be beneficial as we implement robust type checks to ensure data integrity.
Setting Up Your Development Environment
Your first step is to set up a React application. You can utilize Vite as the underlying build tool while installing necessary dependencies such as react-hook-form, Zod, and Tailwind CSS. Tailwind will help create a visually appealing user interface quickly.
Creating Individual Steps
Each step in your multi-step form can function as a standalone component. By utilizing React Hook Form, you can manage individual form field states efficiently. This methodology ensures that validation and user input are streamlined across all steps. For instance:
const Step1 = () => { const { register, handleSubmit } = useFormContext(); const onSubmit = (data) => { console.log(data); }; return (); }
Advantages of Using Context API
The power of React's Context API cannot be understated, especially in multi-step forms. It allows for the full sharing of form state across components without the cumbersome process of prop drilling. Each component can easily access the form state, navigate through the steps, and manage validations seamlessly.
Reducing User Frustration with Data Persistence
A critical aspect of enhancing user experience is preventing data loss during form completion. You can achieve this by persisting the form state in local storage. This way, if a user accidentally refreshes their page, they can continue from where they left off without losing their input.
const saveFormState = () => { localStorage.setItem('formState', JSON.stringify(data)); };
Final Thoughts on Multi-Step Form Design
Building reusable multi-step forms with React Hook Form and Zod represents a powerful way to enhance user interaction with your application. As you implement this, keep the user interface intuitive and engaging, continually iterating based on user feedback. By following best practices in form design, you can significantly improve user engagement and satisfaction, ultimately driving better conversion rates.
Write A Comment