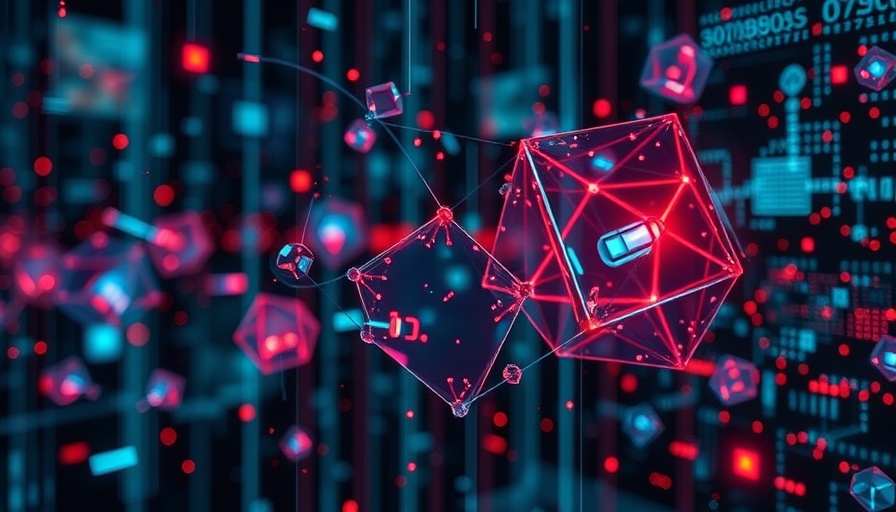
Understanding the Importance of GUIDs/UUIDs
Globally Unique Identifiers (GUIDs) or Universally Unique Identifiers (UUIDs) are critical in software development for ensuring a distinct identification process. With data integrity and uniqueness paramount, utilizing GUIDs/UUIDs can significantly reduce the risks of duplicate entries, especially in large databases. From users to sessions to transaction IDs, every aspect of development can benefit from these identifiers.
The Mechanics of Generating GUIDs in JavaScript
Creating a GUID or UUID in JavaScript is surprisingly straightforward. Most implementations use functions to slice strings and generate characters that comply with the format specified by RFC 4122. The following code snippet illustrates an efficient way to create a UUID:
function generateUUID() { return 'xxxxxxxx-xxxx-4xxx-yxxx-xxxxxxxxxxxx'.replace(/[xy]/g, function(c) { const r = Math.random() * 16 | 0, v = c === 'x' ? r : (r & 0x3 | 0x8); return v.toString(16); });
}
This function provides a full draft of the UUID in the common format, ensuring that it adheres to the standard.
Comparing Different Approaches to Generating UUIDs
While the above method is effective, developers have several libraries available that can simplify this process further. Libraries such as uuid can be leveraged to handle UUID generation effortlessly, emphasizing utility and code readability:
const { v4: uuidv4 } = require('uuid');
console.log(uuidv4());
Utilizing these libraries can streamline development, allowing developers to focus on more pressing code functionality rather than on unique identifier generation.
Common Use Cases for GUIDs/UUIDs in Development
GUIDs and UUIDs are more than just technical necessities; they play integral roles in numerous real-world applications. Some common uses include:
- User IDs in databases - Enables distinct identification of users without conflicts.
- Session tokens - Provides secure session management by ensuring uniqueness between user sessions.
- Transaction IDs - Assures that every transaction can be distinctly recognized for auditing purposes.
Best Practices for Implementing GUIDs/UUIDs
Implementing GUIDs or UUIDs thoughtfully can enhance your coding practices significantly. Here are a few best practices:
- Always opt for UUID version 4 for randomness and security.
- Store GUIDs/UUIDs as strings in your databases to maintain flexibility.
- Regularly audit your implementation to ensure effective usage.
Future Predictions: The Evolution of Identifiers in Web Development
As the web becomes increasingly interconnected, the need for unique identifiers will grow even more critical. Technologies like IoT and distributed ledger currently utilize GUIDs to deal with extensive datasets, but future advancements may introduce even more complex identifier systems that prioritize security and traceability. Keeping abreast of these changes is vital for developers looking to stay ahead.
Concluding Thoughts on GUIDs/UUIDs
Understanding and implementing GUIDs and UUIDs is a fundamental skill for today's web developers. Not only do they ensure data integrity and unique identification, but they also foster better coding practices that can lead to more robust applications. Take the time to integrate these unique identifiers into your coding framework and watch your development processes improve.
Write A Comment